Posted by Armin on Saturday, December 09, 2017
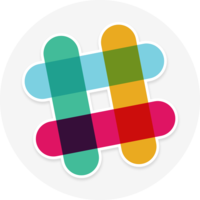
I find Slack extremely useful for collaborating with a team. It's also great for quickly and easily getting notifications about the status of a script that's running on a remote computer somewhere. In my case, I'm running bioinformatics pipelines that take hours or days to run. Slack notifications tell me when programs finish running, or if something odd has happened.
Below are two code snippets I've found useful:
Python class to set up Slack notifications
I wrote the SlackNotifier() class below to send out messages during major steps in my computational pipelines. It gets initialized in the beginning (webhook URL, channel name, etc.), and the "sendMessage()" method gets called every time I want to send a notification to the Slack channel.
import json import requests import socket # just to get hostname. Use os.uname()[1] if already importing os class SlackNotifier(object): """ A class to help send informative messages to our slack channel about the progress of pipeline scripts that can run for hours or days. To use: 1) Turn on "Incoming webhooks" for the slack channel you want: In the Slack web interface, pick a channel and "add an app" Find "Incoming WebHooks" and add it. This will give you the webhook URL to use 2) Import the code here into your project, and then declare a SlackNotifier object, with the webhook and channel name as arguments. 3) Use the sendMessage(msg,title) method to send msg to the channel Example: slack = SlackNotifier("webhook_url_for_slack_api", "channel-name") slack.sendMessage(msg='This is a test message from SlackNotifier()', title='Test message title', subtitle='subtitle', emoji=':computer:') List of emojis can be found here: <a href="https://www.webpagefx.com/tools/emoji-cheat-sheet/ ">https://www.webpagefx.com/tools/emoji-cheat-sheet/ </a> Armin Hinterwirth, 2017 """ def __init__(self, webhook_url, channel_name): self.webhook_url = webhook_url self.channel_name = channel_name self.host_name = socket.gethostname() # Uses the current computers host # name for the header of the message def sendMessage(self, msg, title, subtitle='Message', emoji=":sunglasses:"): """ Send the msg as JSON object to specified channel JSON format found in: <a href="https://github.com/sulhome/bash-slack ">https://github.com/sulhome/bash-slack </a> Emojis: <a href="https://www.webpagefx.com/tools/emoji-cheat-sheet/ ">https://www.webpagefx.com/tools/emoji-cheat-sheet/ </a> """ self.msgdata = { "channel": self.channel_name, "username": self.host_name, "icon_emoji": emoji, "attachments": [ { "fallback": title, "color": "good", "title": title, "fields": [{ "title": subtitle, "value": msg, "short": False }] } ] } # contact the Slack server: response = requests.post( self.webhook_url, data=json.dumps(self.msgdata), headers={'Content-Type': 'application/json'} ) if response.status_code != 200: raise ValueError( 'Request to Slack returned error:\n{}. Response is:\n{}'.format( \ response.status_code, response.text) )
https://bitbucket.org/snippets/amphioxus/yeoREy/slacknotifier-to-send-me...
Get notified on Slack about a change in the IP address of your server
It happens rarely, but sometimes my ISP assigns a new IP address to my home server. If this happened while I'm away, it would be impossible for me to log into my computer. I use the following shell script to get notified of such a change. (I set it up to run once every hour with a cron job.)
#!/usr/bin/env bash # Settings for slack notification: WEBHOOK_URL="THE-URL-YOU-GET-WHEN-SETTING-UP-WEBHOOK-FOR-A-CHANNEL" CHANNEL="channel-name" USERNAME="username" LASTIPFILE='/home/youruser/.last_ip_address'; # Where the last checked IP address is stored # Get public ip address # See: unix.stackexchange.com/a/81699 MYIP=$(dig +short myip.opendns.com @resolver1.opendns.com); LASTIP=$(cat ${LASTIPFILE}); if [[ ${MYIP} != ${LASTIP} ]] then echo "New IP Address = ${MYIP}" echo ${MYIP} > ${LASTIPFILE}; MESSAGETEXT="IP address has changed. It is now: $MYIP" JSON="{\"channel\": \"$CHANNEL\", \"username\":\"$USERNAME\", \"icon_emoji\":\"house\", \"attachments\":[{\"color\":\"danger\" , \"text\": \"$MESSAGETEXT\"}]}" curl -s -d "payload=$JSON" "$WEBHOOK_URL" else echo "IP address (${MYIP}) has not changed." fi